농부지기
2017. 1. 8. 20:41
** 사칙연산(Queue단계별로 보기) **
0. 프로젝트명 : QueueCalculatorStep
1. 사칙연산을 Queue에 넣다 빼면서 처리
2. 화면 설명
- 4칙 연산식 : +-*/ 연산자가 포함된 계산식
- queue1 : 4칙 연산식을 queue에 각 문자열별로 넣은 값
- queue2 : 4칙 연산을 할 때 곱하기, 나누기를 먼저 하고 남은 더하기, 빼기만 남은 연산식
- pop : 아래 [pop]버튼을 클릭 시 queue에서 빼낸 하나의 문자열
- 계산 : 곱셈, 나눗셈 하는 식
- pop버튼 : queue에서 하나를빼내서 보여줌.
3. 소스
https://github.com/farmerkyh/QueueCalculatorStep
4. 화면
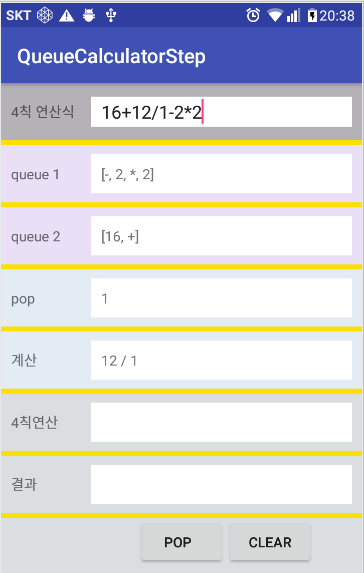
4. 버전
- 안드로이드 스튜디오 : v2.2.3
- Minimum SDK : API 19:Android 4.4 (Kitkat)
5. 소스목록
1. MainActivity.java
2. activity_main.xml
6. 소스
|
1. MainActivity.java |
|
package com.example.farmer.queuecalculatorstep;
import android.graphics.Color; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Gravity; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast;
import java.math.BigDecimal; import java.util.LinkedList; import java.util.Queue;
public class MainActivity extends AppCompatActivity { Queue<String> firstQueue = new LinkedList(); Queue<String> secondQueue = new LinkedList();
TextView tv_queue1; TextView tv_queue2; TextView tv_pop1; TextView tv_pop2; TextView tv_onecalulator; TextView tv_result;
int stepIdx = -1; int seq = 0; String sResult;
@Override protected void onCreate(Bundle savedInstanceState){ super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
//public member 생성 tv_queue1 = (TextView)findViewById(R.id.tv_queue1); tv_queue2 = (TextView)findViewById(R.id.tv_queue2); tv_pop1 = (TextView)findViewById(R.id.tv_pop1); tv_pop2 = (TextView)findViewById(R.id.tv_pop2); tv_onecalulator = (TextView)findViewById(R.id.tv_onecalulator); tv_result = (TextView)findViewById(R.id.tv_result);
//Button Listener 연동 Button btnStep = (Button)findViewById(R.id.btnStep); Button btnClear = (Button)findViewById(R.id.btnClear); btnStep.setOnClickListener(calOnclickListener); btnClear.setOnClickListener(clearOnclickListener); }
Button.OnClickListener calOnclickListener = new View.OnClickListener(){ @Override public void onClick(View view) { stepIdx++; seq = 0; calculatorMain();
//tv_result.setText(sResult); } };
//------------------------------------------------------------------------ // 사칙연사 시작 //------------------------------------------------------------------------ @SuppressWarnings({ "rawtypes", "unchecked" }) public void calculatorMain(){ firstQueue.clear(); secondQueue.clear();
//1. 산술식을 queue에 넣기 stringParsing(); tv_queue1.setText(firstQueue.toString()); if (stepIdx==0) return; //step 1
//2. Queue에 저장 된 값 중 곱셈. 나눗셈 계산 multiplyDivideCal();
//3 Queue에 저장 된 값 중 덧셈. 뺄샘 계산 sResult = addSubtractCal(); //System.out.println("괄호 최종결과 => " + sResult); }
//------------------------------------------------------------------------ // 1차. 문자열 Parsing //------------------------------------------------------------------------ @SuppressWarnings({ "rawtypes", "unchecked" }) public Queue stringParsing(){ String sNumList = ((EditText)findViewById(R.id.et_arithmetic)).getText().toString(); String sOneNum = ""; //하나의 숫자열 for(int i=0; i<sNumList.length(); i++){ String oneChar = sNumList.substring(i, i+1); if ("+-*/()".indexOf(oneChar) >= 0 ){
// 3+(3 과 같이 4칙연산자와 괄호가 동시에 존재 하는 경우 때문 if (!"".equals(sOneNum)) firstQueue.offer(sOneNum); firstQueue.offer(oneChar); sOneNum = ""; }else{ sOneNum += oneChar;
if (i+1 == sNumList.length()){ firstQueue.offer(sOneNum); } } } return firstQueue; }
//------------------------------------------------------------------------ // 곱셈, 나눗셈 계산 //------------------------------------------------------------------------ @SuppressWarnings({ "rawtypes", "unchecked" }) public Queue multiplyDivideCal(){ String oneChar; String sNum1 = "", sNum2 = "", sResult = ""; String operator = ""; BigDecimal nResult = new BigDecimal("0"); while(firstQueue.peek() != null){ oneChar = firstQueue.poll().toString(); seq++; if (stepIdx == seq) tv_queue1.setText(firstQueue.toString()); //step if (stepIdx == seq) tv_pop1.setText(oneChar); //step if (stepIdx == seq) tv_pop2.setText("");
if ("+-".indexOf(oneChar) >= 0 ){ secondQueue.offer(sNum1); secondQueue.offer(oneChar); sNum1 = ""; }else if ("*/".indexOf(oneChar) >= 0 ) { operator = oneChar; }else{ if ("".equals(sNum1)){ sNum1 = oneChar; }else if ("".equals(sNum2)) { sNum2 = oneChar; if ("*".equals(operator)) { nResult = (new BigDecimal(sNum1)).multiply(new BigDecimal(sNum2)); }else if ("/".equals(operator)) { nResult = (new BigDecimal(sNum1)).divide(new BigDecimal(sNum2), 0, BigDecimal.ROUND_UP); }
if (stepIdx == seq) tv_pop2.setText(sNum1 + " " + operator + " " + sNum2); sResult = String.valueOf(nResult);
sNum1 = sResult; sNum2 = ""; operator = ""; } }
if (firstQueue.peek() == null) secondQueue.offer(sNum1);
if (stepIdx == seq) tv_queue2.setText(secondQueue.toString()); //step } return secondQueue; }
//------------------------------------------------------------------------ // 덧셈. 뺄샘 계산 //------------------------------------------------------------------------ @SuppressWarnings({ "rawtypes" }) public String addSubtractCal(){ String operator = ""; String oneChar = ""; //if (secondQueue.peek() == null) return ""; //System.out.println("secondQueue.poll().toString()=" + secondQueue.toString()); BigDecimal nResult = new BigDecimal(secondQueue.poll().toString()); seq++; if (stepIdx == seq) tv_queue2.setText(secondQueue.toString()); //step if (stepIdx == seq) tv_pop1.setText(nResult.toString()); //step if (stepIdx == seq) tv_pop2.setText("");
while(secondQueue.peek() != null){ oneChar = secondQueue.poll().toString(); seq++; if (stepIdx == seq) tv_queue2.setText(secondQueue.toString()); //step if (stepIdx == seq) tv_pop1.setText(oneChar); //step if (stepIdx == seq) tv_pop2.setText("");
if ("+-".indexOf(oneChar) >= 0 ){ operator = oneChar; }else{ if (stepIdx == seq) tv_pop2.setText(nResult + " " + operator + " " + oneChar); if ("+".equals(operator)){ nResult = nResult.add(new BigDecimal(oneChar)); }else if ("-".equals(operator)){ nResult = nResult.subtract(new BigDecimal(oneChar)); } operator = ""; } } return nResult.toString(); }
Button.OnClickListener clearOnclickListener = new View.OnClickListener(){ @Override public void onClick(View view) { stepIdx = -1; seq = 0; tv_queue1.setText(""); tv_queue2.setText(""); tv_pop1.setText(""); tv_pop2.setText(""); tv_onecalulator.setText(""); tv_result.setText("");
//Toast toast = Toast(getApplicationContext()); //toast.setText(sResult); //toast.setDuration(Toast.LENGTH_LONG);
Toast toast = Toast.makeText(getApplicationContext(), sResult, Toast.LENGTH_SHORT); toast.setGravity(Gravity.TOP | Gravity.RIGHT, 0, 0); View viewToast = toast.getView();
//Toast BackgroundColor 변경 //color표기시 xml에서는 "#ffffff"로 하지만 //java코드에서는 아래와 같이 rgb로 변환하여 사용해줘야 한다. viewToast.setBackgroundColor(Color.rgb(255,0,255));
//Toast Font Color변경 TextView tvToast = (TextView)viewToast.findViewById(android.R.id.message); tvToast.setTextColor(Color.RED);
toast.show();
//Toast.makeText(getApplicationContext(), sResult, Toast.LENGTH_LONG).show(); tv_result.setText(sResult); } }; } |
|
2. activity_main.xml |
|
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_keypad" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#fde002" android:orientation="vertical" tools:context="com.example.farmer.queuecalculatorstep.MainActivity">>
<!-- 1차 : 산술식 --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#b4b2b7" android:layout_weight="1">
<TextView android:id="@+id/tv_num" android:padding="10dp" android:layout_width="90dp" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_centerInParent="true" android:text="4칙 연산식"/>
<EditText android:id="@+id/et_arithmetic" android:text="16+12/1-2*2" android:layout_marginRight="10dp" android:layout_width="match_parent" android:layout_height="30dp" android:paddingLeft="10dp" android:background="#ffffff" android:layout_centerInParent="true" android:layout_toRightOf="@+id/tv_num" />
</RelativeLayout>
<!-- 2차 : queue1 --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#e9e0f7" android:layout_marginTop="5dp" android:layout_weight="1">
<TextView android:id="@+id/tv_lavel1" android:padding="10dp" android:layout_width="90dp" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_centerInParent="true" android:text="queue 1"/>
<TextView android:id="@+id/tv_queue1" android:padding="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:paddingLeft="10dp" android:layout_marginRight="10dp" android:layout_centerInParent="true" android:layout_toRightOf="@+id/tv_lavel1" android:background="#ffffff" android:text="queue 1"/> </RelativeLayout>
<!-- 3차 : queue2 --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#e9e0f7" android:layout_marginTop="5dp" android:layout_weight="1">
<TextView android:id="@+id/tv_lavel2" android:padding="10dp" android:layout_width="90dp" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_centerInParent="true" android:text="queue 2"/>
<TextView android:id="@+id/tv_queue2" android:padding="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:paddingLeft="10dp" android:layout_marginRight="10dp" android:layout_centerInParent="true" android:layout_toRightOf="@+id/tv_lavel2" android:background="#ffffff" android:text="queue 2"/> </RelativeLayout>
<!-- 5차 : pop1 --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#e3ebf4" android:layout_marginTop="5dp" android:layout_weight="1">
<TextView android:id="@+id/tv_poplavel1" android:padding="10dp" android:layout_width="90dp" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_centerInParent="true" android:text="pop"/>
<TextView android:id="@+id/tv_pop1" android:padding="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:paddingLeft="10dp" android:layout_marginRight="10dp" android:layout_centerInParent="true" android:layout_toRightOf="@+id/tv_poplavel1" android:background="#ffffff" android:text=""/> </RelativeLayout>
<!-- 5차 : pop2 --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#e3ebf4" android:layout_marginTop="5dp" android:layout_weight="1">
<TextView android:id="@+id/tv_poplavel3" android:padding="10dp" android:layout_width="90dp" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_centerInParent="true" android:text="계산"/>
<TextView android:id="@+id/tv_pop2" android:padding="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:paddingLeft="10dp" android:layout_marginRight="10dp" android:layout_centerInParent="true" android:layout_toRightOf="@+id/tv_poplavel3" android:background="#ffffff" android:text=""/> </RelativeLayout>
<!-- 6차 : 4칙연산 하나 계산 --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#dbdee1" android:layout_marginTop="5dp" android:layout_weight="1">
<TextView android:id="@+id/tv_onelavel" android:padding="10dp" android:layout_width="90dp" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_centerInParent="true" android:text="4칙연산"/>
<TextView android:id="@+id/tv_onecalulator" android:padding="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:paddingLeft="10dp" android:layout_marginRight="10dp" android:layout_centerInParent="true" android:layout_toRightOf="@+id/tv_onelavel" android:background="#ffffff" android:text=""/> </RelativeLayout>
<!-- 7차 : 최종 계산 --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#dbdee1" android:layout_marginTop="5dp" android:layout_weight="1">
<TextView android:id="@+id/tv_resultlabel" android:padding="10dp" android:layout_width="90dp" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_centerInParent="true" android:text="결과"/>
<TextView android:id="@+id/tv_result" android:padding="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:paddingLeft="10dp" android:layout_marginRight="10dp" android:layout_centerInParent="true" android:layout_toRightOf="@+id/tv_resultlabel" android:background="#ffffff" android:text=""/> </RelativeLayout>
<!-- 8차 : step --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#dbdee1" android:layout_marginTop="5dp" android:layout_weight="1">
<Button android:id="@+id/btnStep" android:text="POP" android:layout_centerHorizontal="true" android:layout_width="wrap_content" android:paddingRight="20dp" android:layout_height="wrap_content" />
<Button android:id="@+id/btnClear" android:text="Clear" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_toRightOf="@id/btnStep" /> </RelativeLayout>
<!-- 9차 : 빈공백 --> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:background="#ffffff" android:layout_weight="0.5"> </RelativeLayout> </LinearLayout> |